SDK functions¶
This chapter explains the functions you can use when developing your own application or plugin. Below figure illustrates the project structure of ViCANdo:
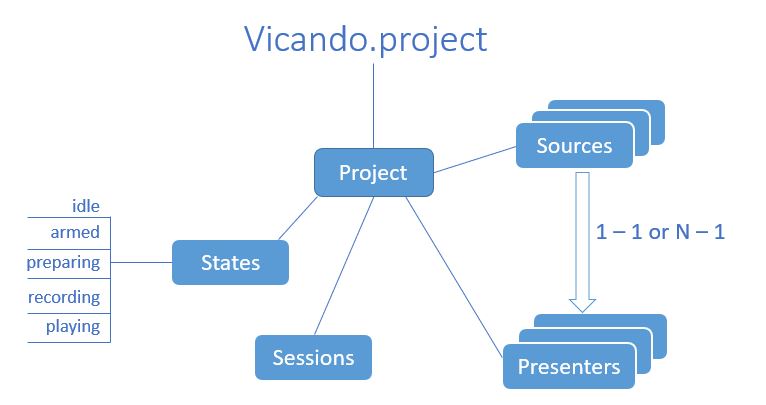
vxobject.h¶
VxObject¶
-
class
VxObject
: public QObject¶
Constructs an object:
-
explicit
VxObject
()
Increases the reference count of object:
-
void
ref
()¶
Increase the reference count of object, and possibly remove the floating reference, if object has a floating reference:
-
void
sinkRef
()¶
Decreases the reference count of object. When the reference count is 0, the object is finalized(i.e. its memory is freed):
-
void
unref
()¶
Removes the floating reference of the object(Sets the floating state to false):
-
void
sink
()¶
Checks whether object has a floating reference:
-
bool
isFloating
()¶
Gets descriptive name that can be shown in the project explorer:
-
virtual const QString
getObjectText
()¶
Returns the reference count of object:
-
int
refCount
()¶
VxReference¶
-
template<VxReference
T
>
classVxReference
¶
Constructs an object with no references:
-
VxReference
()¶
Constructs an object with reference copied from the input _copy:
-
VxReference
(const VxReference<T> &_copy)¶
Constructs an object with an object pointer:
-
VxReference
(T *_object_ptr)¶
Destructor:
-
~VxReference
()¶
Takes the reference count from the input _object:
-
void
take
(T *_object)¶
Gets the current reference count of the object:
-
T *
get
() const¶
The operator to get a member:
-
T *
operator->
()¶
The operator to get the pointer of member:
-
const T *
operator->
() const¶
The operator to get the pointer of a member:
-
operator T*
() const¶
The operator to get the address of the member:
-
T *
operator&
() const¶
Equal operator:
-
VxReference<T> &
operator=
(const VxReference<T> &_copy)¶
Equal operator:
-
VxReference<T> &
operator=
(T *object_ptr)¶
Other functions¶
Returns the hash code of the VxReference object:
-
inline uint
qHash
(VxReference<T> object)¶
vxdata.h¶
Property |
type |
READ accessor |
---|---|---|
time |
qint64 |
getTimeStampInUs() |
data |
QVariantList |
getVariantData() |
Constructs a VxData object with argument timestamp in micro-seconds:
-
VxData
(qint64 _time_stamp_in_us)
Converts to QByteArray:
-
virtual QByteArray
toByteArray
()¶
Returns true if objects are equal:
Converts QScriptEngine object to QScriptValue:
-
virtual QScriptValue
toScriptValue
(QScriptEngine *script_engine)¶
Retrives the DataRowRenderer:
-
virtual VxDataRowRenderer *
getDataRowRenderer
()¶
Returns the value of the property time:
-
qint64
getTimeStampInUs
()¶
Returns true if the object is statistic data:
-
bool
isStatisticData
()¶
Returns the value of the property data:
-
QVariantList
getVariantData
()¶
vxapprt.h¶
This file contains macro definitions for the shared library
vxtimer.h¶
Constructs a VxTimer object:
-
VxTimer
()
Gets descriptive name that can be shown in the project explorer:
-
const QString
getObjectText
()
Restarts the timer:
-
void
restart
()¶
Sets the offset time in milli-seconds:
-
void
setTimeOffsetInMs
(qint64 time_in_ms)¶
Sets the offset time in micro-seconds:
-
void
setTimeOffsetInUs
(qint64 time_in_us)¶
Returns the elapsed time in milli-seconds:
-
qint64
elapsedInMs
()¶
Returns the elapsed time in micro-seconds:
-
qint64
elapsedInUs
()¶
Returns the current timestamp in milli-seconds:
-
static qint64
getCurrentTimeInMs
()¶
Returns the current timestamp in micro-seconds:
-
static qint64
getCurrentTimeInUs
()¶
vxcomponent.h¶
Property |
type |
READ accessor |
---|---|---|
dragEnabled |
int |
isDragEnabled() |
dropEnabled |
int |
isDropEnabled() |
Constructs a VxComponent object:
-
VxComponent
()
Public functions¶
Get Icon to display:
-
virtual QIcon
getIcon
()¶
Returns the number of the properties:
-
int
getPropertyCount
()¶
Returns the property descriptor of the component based on the index:
-
VxReference<VxPropertyDescriptor>
getPropertyDescriptor
(int index)¶
Sets the component object ID, this method should only be called once:
-
void
setObjectID
(const quint64 &_object_id)¶
Retrieves the component object ID. This method may not be invoked before setObjectID has been invoked:
-
quint64
getObjectID
()¶
Returns true if object ID is already set by setObjectID():
-
bool
isObjectIDSet
()¶
Actions to display in context menu for component:
-
virtual QList<QAction*>
actions
(QWidget *parent)¶
Register source to the script engine. Returns the object that will be registered to the script engine:
-
virtual QScriptValue
registerToScriptEngine
(QScriptEngine *_script_engine)¶
Drag and Drop. Returns true if drag is enabled for this component:
-
bool
isDragEnabled
()¶
Drag and Drop. Returns true if drop is enabled for this component:
-
bool
isDropEnabled
()¶
Returns true if the mime_data can be handled by the model, otherwise returns false:
Signals¶
New message to display on the console:
-
void
newLogMessage
(const QString &message)¶
Information for the object is updated:
Emitted when a property for this has changed:
-
void
propertyChanged
(const QString &property_name)¶
Emitted if user wants to delete this compoent:
-
void
deleteComponent
(VxComponent *_this)¶
Emitted when all properties in projects need to be refreshed:
-
void
refreshAllProperties
()¶
Public slots¶
Write a new message to the console windows:
-
void
logMessage
(const QString &message)¶
Deletes the component:
-
void
deleteComponentAction
()¶
Protected functions¶
Invoked when user request this component to be deleted. Optionally implemented by subclasses to add some special handling to this event:
-
virtual void
componentDeleteRequest
()¶
Add a new property descriptor to the list:
-
void
addProperty
(VxPropertyDescriptor *property_descriptor)¶
Remove the component property:
-
void
removeComponentProperty
(const QString &property_name)¶
Reference to the script engine used by the project. Returns 0 before registerToScriptEngine has been invoked:
-
QScriptEngine *
scriptEngine
()¶
Every component has an object ID that is unique within the project:
-
quint64
object_id
¶
Enable/Disable drag for this component:
-
void
setDragEnabled
(bool enabled)¶
Enable/Disable drop for this component:
-
void
setDropEnabled
(bool enabled)¶
Other functions¶
Returns the hash code of the object: .. cpp:function:: inline uint qHash(VxReference<VxComponent> component)
vxappqmlapplicationengine.h¶
Main function¶
The main function of the application. You can specify the arguments, application icon file and your own application engine object:
-
int
vxAPP_main
(int argc, char *argv[], const QString &app_icon, const QMetaObject *app_engine_class)¶
VxAppQmlApplicationEngine¶
To be able to understand below functions, you need to have basic knowledge of QML/C++ hybrid programming skills. Below links from Qt provide useful information for you to get started. You can also refer to the simple J1939 demo application source code to learn how to setup your own QML application on top of the ViCANdo project.
VxAppQmlApplicationEngine class provides a convenient way to load an application from the single qml file:
-
class
VxAppQmlApplicationEngine
: public QQmlApplicationEngine¶
Parameterized Constructor. Parameter _app_name is your application’s name. Parameter _project_path is the path of the ViCANdo.project file inside your Qt application:
-
VxAppQmlApplicationEngine
(const QString &_app_name, const QString &_project_path)
Class destructor:
-
~VxAppQmlApplicationEngine
()
Virtual initialization function. You need to implement your own init() function:
-
virtual void
init
()¶
Virtual postInit() function. To be executed after init() was called:
-
virtual void
postInit
()¶
Checks the ViCANdo SDK license status of the connected USB-CAN interface. Return false if the license is invalid:
-
bool
checkLicense
()¶
Updates the list of the available CAN channels:
-
void
updateCANChannelList
()¶
Returns the pointer of the QML component object:
-
QQmlComponent *
getQMLComponent
()¶
Sets the project recording_directory path:
-
void
setProjectRecordingDirectory
(const QString &recording_directory_path)¶
Finds the object in the project by the object name which can be set in the Component properties window of the sources in ViCANdo. Returns the pointer of the object:
-
QObject *
findProjectObject
(const QString &component_name)¶
vxproject.h¶
VxProject class is the base class of the ViCANdo project. From this class you will have access to the properties and configuration of the project.
-
class
VxProject
: public VxComponent¶
Property |
type |
READ accessor |
---|---|---|
projectName |
QString |
getProjectName() |
preTriggerTimeInMs |
int |
getPreTriggerTime() |
limitRecordTime |
bool |
getLimitRecordTime() |
returnToArmedAfterTrigger |
bool |
getReturnToArmedAfterTrigger() |
maxRecordingTimeInS |
int |
getMaxRecordingTime() |
maxRecordingTimeInSVisible |
bool |
getLimitRecordTime(). True if limit record time is checked |
continueRecordInANewSession |
bool |
getContinueRecordInANewSession() |
continueRecordInANewSessionVisible |
bool |
getLimitRecordTime(). True if limit record time is checked |
Public functions¶
Class constructor:
-
VxProject
(const QString &name, const QString &directory)
Arguments:
name is the name of the project.
directory is the folder directory of the project.
Open project dialog:
-
static QString
openProjectDialog
(QWidget *parent = 0)¶
Open project:
-
static VxReference<VxProject>
openProject
(const QString &project_path, QWidget *parent = 0)¶
Arguments:
project_path is the absolute path of the project.
Save project:
-
void
saveProject
()¶
Load project:
-
bool
loadProject
()¶
Save project as:
-
void
saveProjectAs
(QWidget *parent)¶
Get next object ID:
-
quint64
nextObjectID
()¶
State¶
Enumerator State provides all kinds of states the project can have, corresponding with the menus in the software.
Idle state is when a project is opened but no action were taken.
-
enumerator
State
::
Idle
¶
-
enumerator
State
::
WaitingForPrepare
¶
-
enumerator
State
::
Prepare
¶
Recording state is when the record button is clicked. ViCANdo starts to record the data.
-
enumerator
State
::
Recording
¶
Playing state is when a session is selected to playback.
-
enumerator
State
::
Playing
¶
Pause state is when pause menu is clicked.
-
enumerator
State
::
Pause
¶
-
enumerator
State
::
RewindingBeforePlayback
¶
TriggerAction¶
Enumerator TriggerAction provides the default actions for a trigger.
This action will start recording when the trigger is trigged:
-
enumerator
TriggerAction
::
StartRecordAction
¶
This action will stop recording when the trigger is trigged:
-
enumerator
TriggerAction
::
StopRecordAction
¶
This action will insert a log marker when the trigger is trigged:
-
enumerator
TriggerAction
::
LogMarkerAction
¶
This action will set a bookmark when the trigger is trigged:
-
enumerator
TriggerAction
::
SetBookmarkAction
¶
-
const QString
getObjectText
()
-
QList<QAction*>
actions
(QWidget *parent)
-
bool
isReadOnlyResource
()¶
-
const QString &
getDirectory
()¶
-
const QString &
getRecordDirectory
()¶
-
void
setProjectRecordDirectory
(const QString &_project_record_directory)¶
-
const QString &
getApplicationDirectory
()¶
-
void
setApplicationDirectory
(const QString &app_dir)¶
-
QList<VxReference<VxDataSource>>
getDataSourceList
()¶
-
QList<VxReference<VxSlaveSource>>
getSlaveSourceList
()¶
-
QList<VxReference<VxSource>>
getSourceList
()¶
-
int
getDataSourceCount
()¶
-
QList<VxReference<VxPresenter>>
getPresenterList
()¶
-
QList<VxReference<VxTrigger>>
getTriggerList
()¶
-
QList<VxReference<VxScriptletComponent>>
getScriptletList
()¶
-
QList<VxReference<VxDBCComponent>>
getDBCList
()¶
-
VxReference<VxDBCComponent>
getDBCComponentById
(quint64 component_id)¶
-
VxReference<VxDBCComponent>
getDBCComponentByName
(const QString &name)¶
-
VxReference<VxDBCComponent>
getDBCComponentByFilePath
(const QString &file_path)¶
-
VxReference<VxScriptletComponent>
getScriptletByName
(const QString &name)¶
-
QStringList
getTriggerActionTextList
()¶
-
const QString
getProjectName
()¶
-
void
setProjectName
(const QString _project_name)¶
-
int
getPreTriggerTime
()¶
-
void
setPreTriggerTime
(int pre_trigger_time_in_ms)¶
-
qint64
getRecordTimerStartOffsetInUs
()¶
-
bool
getReturnToArmedAfterTrigger
()¶
-
void
setReturnToArmedAfterTrigger
(bool v)¶
-
bool
getLimitRecordTime
()¶
-
void
setLimitRecordTime
(bool v)¶
-
void
resetRecordTimer
()¶
-
int
getMaxRecordingTime
()¶
-
void
setMaxRecordingTime
(int max_recording_time_in_s)¶
-
bool
getContinueRecordInANewSession
()¶
-
void
setContinueRecordInANewSession
(bool v)¶
-
QList<VxReference<VxDataSource>>
getDataSourceListMatchingType
(const QMetaObject *meta_object)¶
-
QList<VxReference<VxDataSource>>
getDataSourceListMatchingType
(const char *class_name)¶
template<class T> .. cpp:function:: QList<VxReference<T> > getDataSourceListMatchingType()
template<class T> .. cpp:function:: QList<VxReference<T> > getPresenterListMatchingType()
template<class T> .. cpp:function:: QList<VxReference<T> > getPresenterListMatchingType(const QString& type_name)
template<class T> .. cpp:function:: QList<VxReference<VxPresenter> > getPresenterListMatchingInterface()
-
QList<VxReference<VxSession>>
getAllSessionList
()¶
-
QList<VxReference<VxSessionGroup>>
getTopLevelSessionGroupList
()¶
-
VxReference<VxSessionGroup>
getAllSessionsGroup
()¶
-
VxReference<VxSession>
getSessionByID
(quint64 object_id)¶
-
void
addNewSession
(VxReference<VxSession> session)¶
-
void
addNewSessionGroup
(VxReference<VxSessionGroup> session_group, VxSessionGroup *parent_session_group = 0)¶
-
int
addSessionGroup
(VxReference<VxSessionGroup> session_group, VxSessionGroup *parent_session_group)¶
-
void
enumerateSessionGroups
(const QString &name)¶
-
State
getCurrentState
()¶
-
VxSession *
getCurrentSession
()¶
-
VxScriptEngine *
getScriptEngine
()¶
-
VxReference<VxMarkerList>
getMarkerList
()¶
-
VxReference<VxDataSource>
getDataSourceMatching
(quint64 source_id)¶
-
VxReference<VxSource>
getSourceMatching
(quint64 source_id)¶
-
VxReference<VxPresenter>
getPresenterMatching
(quint64 source_id)¶
-
VxReference<VxLogDataFile>
getLogDataFileMatching
(quint64 log_data_file_id)¶
-
void
addDataSource
(VxDataSource *source)¶
-
void
addSlaveSource
(VxSlaveSource *source)¶
-
void
addPresenter
(VxPresenter *presenter)¶
-
void
addTrigger
(VxTrigger *trigger)¶
-
void
addScriptlet
(VxScriptletComponent *scriptlet)¶
-
void
addDBC
(VxDBCComponent *dbc_component)¶
-
bool
prepareSession
()¶
-
bool
startRecord
()¶
-
bool
breakRecord
(bool create_new_session)¶
-
bool
playSession
(VxSession *session)¶
-
bool
seekToPositionForSession
(VxSession *session, qint64 position_in_time)¶
-
bool
pause
()¶
-
bool
resume
()¶
-
void
stop
(bool called_from_scriplet = false)¶
-
void
shutdown
(bool called_from_scriplet = false)¶
-
VxDataSource::PlaybackMode
getPlaybackMode
()¶
-
void
setPlaybackMode
(VxDataSource::PlaybackMode _playback_mode)¶
-
QIcon
getIcon
()
-
void
clearAppicationSettings
()¶
-
void
addApplicationSetting
(const QString &key, const QByteArray value)¶
-
QByteArray
getApplicationSetting
(const QString &key)¶
-
bool
hasApplicationSettingKey
(const QString &key)¶
-
void
emitNewSearchMatch
(VxSession *session, VxDataSource *source, qint64 time_pos, const QString data_content_text)¶
-
void
emitSessionRangeChanged
(VxSession *session)¶
-
VxReference<VxSlaveSource>
getSlaveSourceFor
(VxComponent *originator, VxSource *master_source, const QString &key, qint64 object_id = -1)¶
-
void
invalidateSlaveSourcesFor
(VxComponent *originator)¶
-
void
removeInvalidSlaveSources
()¶
-
VxReference<VxComponent>
getOriginatorFor
(VxReference<VxSlaveSource> slave_source)¶
-
void
detachSlaveSource
(VxPresenter *from_presenter, VxSlaveSource *slave_source)¶
-
void
detachTrigger
(VxTrigger *trigger)¶
-
VxProjectQMLProxy *
getQMLProxy
()¶
-
void
emitClearConsoleSignal
()¶
-
void
removeDataSource
(VxDataSource *data_source)¶
-
void
removeComponentFromProject
(VxComponent *component)¶
-
void
storeScriptValue
(const QString &key, const QString &value)¶
-
QString
readStoredScriptValue
(const QString &key)¶
-
void
removeStoredScriptValue
(const QString &key)¶
-
bool
hasStoredScriptValue
(const QString key)¶
-
void
clearStoredScriptValues
()¶
-
VxReference<VxTimerBase>
getRecordTimer
()¶
-
VxReference<VxTimerBase>
getPlaybackTimer
()¶
-
float
getPlaybackFactor
()¶
-
void
selectPresenter
(VxPresenter *presenter)¶
-
QString
getRecordStartStopReason
()¶
-
QList<VxReference<VxBookmark>>
getRecordSessionBookmarkList
()¶
Signals¶
-
void
newSourceAdded
(VxSource *source)¶
-
void
newPresenterAdded
(VxPresenter *presenter)¶
-
void
newSessionGroupAdded
(VxSessionGroup *session_group, int index)¶
-
void
newTriggerAdded
(VxTrigger *data_trigger)¶
-
void
newScriptletAdded
(VxScriptletComponent *scriptlet)¶
-
void
newDBCAdded
(VxDBCComponent *dbc)¶
-
void
currentSessionChanged
(VxSession *session)¶
-
void
componentRemoved
(VxComponent *component)¶
-
void
playTimeUpdated
(qint64 elapsed_time_in_us, qint64 end_time_in_us)¶
-
void
startSeek
()¶
-
void
seekDone
()¶
-
void
clearSelection
()¶
-
void
clearSearchResults
()¶
-
void
searchDone
()¶
-
void
newSearchMatch
(VxSession *session, VxDataSource *source, qint64 time_pos, const QString &data_content_text)¶
-
void
clearConsole
()¶
-
void
playbackFinished
()¶
-
void
playbackModeChanged
(VxDataSource::PlaybackMode playback_mode)¶
-
void
sessionRangeChanged
(VxSession *session)¶
-
void
updateSession
(VxSession *session)¶
-
void
projectNameChanged
()¶
-
void
recordBreak
(qint64 break_time_in_us)¶
Public slots¶
-
void
setPlaybackSpeedFactor
(float factor)¶
-
void
updatePlaytime
()¶
-
void
openSearchDialog
(VxSessionGroup *session_group, VxSession *session)¶
-
void
setHighlightedText
(const QString &text, Qt::CaseSensitivity case_sensitivity = Qt::CaseInsensitive)¶
-
void
clearPresenterViews
()¶
-
void
startBacktraceUpdate
()¶
-
void
stopBacktraceThread
()¶
-
void
restartScriptEngine
()¶
-
void
mark
(const QString &marker_id)¶
vxsource.h¶
TODO
vxpresenter.h¶
TODO
vxqueue.h¶
TODO
vxtimebase.h¶
TODO
vxcanflags.h¶
A class contains all the CAN related enumerations.
-
class
VxCANFlags
¶
MessageFlagsMask¶
MessageFlagsMask provides the message flags. All flags and/or combinations of them are meaningful for both transmitted and received CAN messages:
-
enumerator
MessageFlagsMask
::
Rtr
= 0x00001¶
-
enumerator
MessageFlagsMask
::
Standard
= 0x00002¶
-
enumerator
MessageFlagsMask
::
Extended
= 0x00004¶
-
enumerator
MessageFlagsMask
::
Wakeup
= 0x00008¶
-
enumerator
MessageFlagsMask
::
NError
= 0x00010¶
-
enumerator
MessageFlagsMask
::
ErrorFrame
= 0x00020¶
-
enumerator
MessageFlagsMask
::
TxMsgAcknowledge
= 0x00040¶
-
enumerator
MessageFlagsMask
::
TxMsgRequest
= 0x00080¶
-
enumerator
MessageFlagsMask
::
ErrorMask
= 0x0ff00¶
-
enumerator
MessageFlagsMask
::
ErrorHWOverrun
= 0x00200¶
-
enumerator
MessageFlagsMask
::
ErrorSWOverrun
= 0x00400¶
-
enumerator
MessageFlagsMask
::
ErrorStuff
= 0x00800¶
-
enumerator
MessageFlagsMask
::
ErrorForm
= 0x01000¶
-
enumerator
MessageFlagsMask
::
ErrorCRC
= 0x02000¶
-
enumerator
MessageFlagsMask
::
ErrorBIT0
= 0x04000¶
-
enumerator
MessageFlagsMask
::
ErrorBIT1
= 0x08000¶
-
enumerator
MessageFlagsMask
::
InternalFrame
= 0x10000¶
-
enumerator
MessageFlagsMask
::
ISO15765ExtAddr
= 0x20000¶
-
enumerator
MessageFlagsMask
::
ISO15765UnknownType
= 0x40000¶
CapabilitesMask¶
CapabilitesMask provides additional function masks for the messages. It specifies the type of the message:
-
enumerator
CapabilitesMask
::
ExtendedCAN
= 0x00000001L¶
-
enumerator
CapabilitesMask
::
BusStatistics
= 0x00000002L¶
-
enumerator
CapabilitesMask
::
ErrorCounters
= 0x00000004L¶
-
enumerator
CapabilitesMask
::
CanDiagnostics
= 0x00000008L¶
-
enumerator
CapabilitesMask
::
GenerateError
= 0x00000010L¶
-
enumerator
CapabilitesMask
::
GenerateOverload
= 0x00000020L¶
-
enumerator
CapabilitesMask
::
TxRequest
= 0x00000040L¶
-
enumerator
CapabilitesMask
::
TxAcknowledge
= 0x00000080L¶
-
enumerator
CapabilitesMask
::
Virtual
= 0x00010000L¶
-
enumerator
CapabilitesMask
::
Simulated
= 0x00020000L¶
-
enumerator
CapabilitesMask
::
Remote
= 0x00040000L¶
DriverMode¶
DriverMode provides the general CAN bus driver mode.
-
enumerator
DriverMode
::
Off
= 0¶
-
enumerator
DriverMode
::
Silent
= 1¶
-
enumerator
DriverMode
::
Normal
= 4¶
-
enumerator
DriverMode
::
SelfReception
= 8¶
ReadResult¶
ReadResult provides the status code for the function that reads the CAN message:
-
enumerator
ReadResult
::
ReadStatusOK
= 0¶
-
enumerator
ReadResult
::
ReadTimeout
= -1¶
-
enumerator
ReadResult
::
ReadError
= -2¶
SendResult¶
SendResult provides the status code for the function that transmits the CAN message:
-
enumerator
SendResult
::
SendStatusOK
= 0¶
-
enumerator
SendResult
::
SendTimeout
= -1¶
-
enumerator
SendResult
::
TransmitBufferOveflow
= -2¶
-
enumerator
SendResult
::
SendInvalidParam
= -3¶
-
enumerator
SendResult
::
SendError
= -4¶
vxcaninterface.h¶
An interface class that describles general functions needed for supported CAN interfaces:
-
class
VxCANInterface
: public VxCANFlags¶
Class constructor:
-
VxCANInterface
()
Returns the capabilite of the message. The return value can be one or combined CapabilitesMask.
-
virtual quint32
canGetCapabilites
()¶
Reads a CAN message from the receive buffer of the CAN interface:
-
virtual ReadResult
canRead
(long &id, QByteArray &data, unsigned int &flag, qint64 ×tmap_in_us, int timeout_in_ms)¶
Arguments:
id is the CAN message identifier.
data is the data field of the CAN message.
flag is one or combined MessageFlagsMask.
timestmap_in_us is the timestamp of the message in micro-seconds.
timeout_in_ms is the maximum time in milli-seconds to read the message. If no message is read when time reachs timeout_in_ms, ReadResult::ReadTimeout will be returned.
Returns: One of the ReadResult.
Sends a CAN message from the CAN interface:
-
virtual SendResult
canSend
(const long id, const QByteArray &data, const unsigned int flag, int timeout_in_ms)¶
Arguments:
id is the CAN message identifier.
data is the data field of the CAN message.
flag is one or combined MessageFlagsMask.
timeout_in_ms is the maximum time in milli-seconds to send the message. If no message is sent when time reachs timeout_in_ms, SendResult::SendTimeout will be returned.
Returns: One of the SendResult.
vxplugindescriptor.h¶
-
class
VxPluginDescriptor
: public QObject¶
Class constructor:
-
VxPluginDescriptor
(const QString &_name, const QString &_description)
Arguments:
_name is the name of the plugin.
_description is the description of the plugin.
Returns the name of the plugin:
-
const QString &
getName
()¶
Returns the description of the plugin:
-
const QString &
getDescription
()¶
Register as the global resources:
-
virtual void
registerGlobalResources
()¶
vxvideo.h¶
This file contains macro definitions for the video module.
vxvideoframedata.h¶
Open CV , the open source computer vision and machine learning software library was used in ViCANdo SDK. This class provides the functions for processing video frame data.
Class constructor:
-
VxVideoFrameData
(int _frame_number, qint64 _time_stamp)
Arguments:
_frame_number is the number of the video frame.
_time_stamp is the timestamp of the video frame.
Returns the IplImage format of the video frame. Read more about IplImage from this link
-
virtual IplImage *
getIplImage
()¶
Returns Scriptvalue of the script engine:
-
QScriptValue
toScriptValue
(QScriptEngine *script_engine)
Converts the video frame to QImage:
-
QImage
toQImage
()¶
Returns the frame number:
-
int
getFrameNumber
() const¶
Returns the maximum length of the time format. The time format is 00:00:00.000, with a length of 12.
-
static int
getTimeFormatMaxLength
()¶
Returns the application timer format:
-
QString
getApplicationTimerFormat
()¶
Returns the format of the frame count:
-
QString
getFrameCountFormat
()¶
Returns true if two VxData objects are the same:
-
bool
equals
(VxData *data)
vximageprocessor.h¶
Nested class: Object¶
-
class
Object
¶
Members:
-
QPen
pen
¶
-
QPolygon
polygon
¶
-
QString
info
¶
-
QPoint
info_pos
¶
Resizes the polygon:
-
QPolygon
scale
(const QSize &from_size, const QSize &to_size)¶
Arguments:
from_size is the original size of the polygon.
to_size is the destination size you want to resize to.
Resizes the point:
-
QPoint
scaleInfoPos
(const QSize &from_size, const QSize &to_size)¶
Arguments:
from_size is the original size of the point.
to_size is the destination size you want to resize to.
Public functions¶
Class constructor:
-
VxImageProcessor
()
Processes the input video frame data:
-
virtual void
processVideoFrameData
(VxReference<VxVideoFrameData> _video_frame_data)¶
Signals¶
Updates the object list:
-
void
updateObjectList
(const QString &key, QList<VxImageProcessor::Object> object_list)¶
Arguments:
key is the key of the object list to be updated.
object_list is the object list to be updated.
vximageprocessordescriptor.h¶
-
class
VxImageProcessorDescriptor
: public VxPluginDescriptor¶
Class constructor:
-
VxImageProcessorDescriptor
(const QString &_name, const QString &_description)
Arguments:
_name is the name of the image processor.
_description is the description of the image processor.
Class destructor:
-
~VxImageProcessorDescriptor
()
Creates a VxImageProcessor object for the input VxProject object:
-
virtual VxImageProcessor *
create
(VxProject *project)¶
Loads the VxImageProcessor object:
-
virtual VxImageProcessor *
load
(VxProject *project, QDomElement &source_root)¶
Arguments:
project is the pointer of the VxProject object.
source_root is the address of the element in the DOM tree.
vximagemathutils.h¶
This file contains the utility classes of the image math calculations.
VxExpMovingAverage¶
A class handles the exponential moving average(EMA)
-
class
VxExpMovingAverage
¶
Class constructor:
-
VxExpMovingAverage
()
Clears current EMA:
-
void
clear
()¶
Adds value to current EMA:
-
void
add
(double value)¶
Gets the current EMA:
-
double
get
()¶
VxImageMath¶
-
class
VxImageMath
¶
Returns the subtraction of two 2D 32-bit floating points:
-
static CvPoint2D32f
sub
(CvPoint2D32f b, CvPoint2D32f a)¶
Returns the multiplication of two 2D 32-bit floating points:
-
static CvPoint2D32f
mul
(CvPoint2D32f b, CvPoint2D32f a)¶
Returns the addition of two 2D 32-bit floating points:
-
static CvPoint2D32f
add
(CvPoint2D32f b, CvPoint2D32f a)¶
Multiplies a point by a scalar:
-
static CvPoint2D32f
mul
(CvPoint2D32f b, float t)¶
Arguments:
b is the 2D point.
t is the scalar.
Returns the Dot Product of two points:
-
static float
dot
(CvPoint2D32f a, CvPoint2D32f b)¶
Returns the distance of the point v:
-
static float
dist
(CvPoint2D32f v)¶
Gets the closest point on segment:
-
static CvPoint2D32f
pointOnSegment
(CvPoint2D32f line0, CvPoint2D32f line1, CvPoint2D32f pt)¶
Arguments:
line0 is one end point coordinate of the segment.
line1 is the other end point coordinate of the segment.
pt is any point on the segment.
Returns the perpendicular distance between point and line:
-
static float
dist2line
(CvPoint2D32f line0, CvPoint2D32f line1, CvPoint2D32f pt)¶
Arguments:
line0 is one end point coordinate of the line.
line1 is the other end point coordinate of the line.
pt is the point.